本教程只基于Formily SchemaForm的使用,Demo展示 codesandbox
Formily是什么
Formily 是阿里巴巴开源的一个表单框架,致力于解决所有中后台表单场景。目前只有React版本,支持Antd和next UI组件库。[查看详情]
Formily有自己的状态管理,内部也有自己的生命周期,在渲染的时候也做到了最小更新,所以即使你是大型表单性能也不会很差。
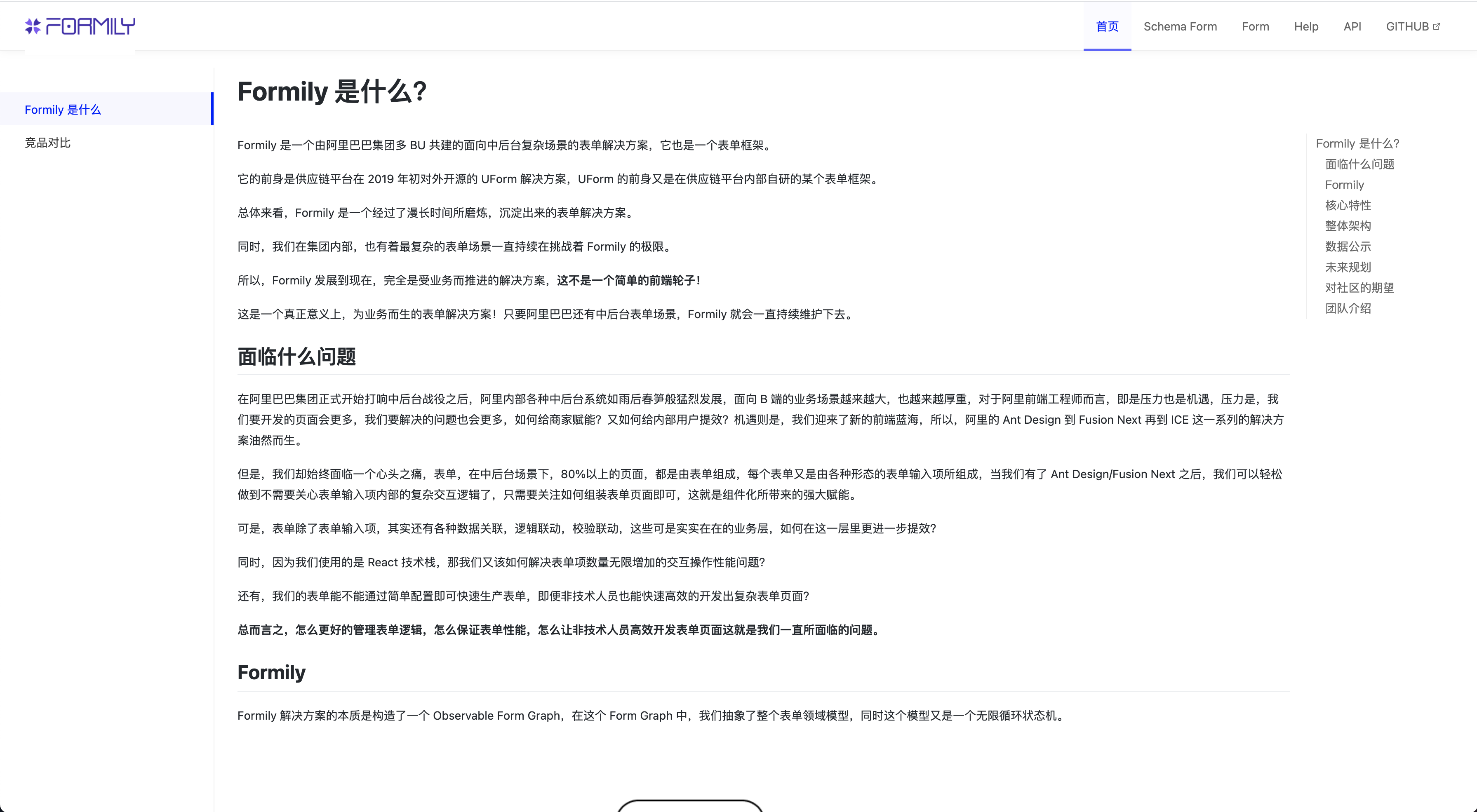
SchemaMarkupField - 用于描述表单字段 [查看详情]
使用Formily实现一个基础表单
这是一个使用 SchemaForm 实现的一个最简单的表单,里面有一个nickname字段,当用户点击提交的时候,会触发 SchemaForm 的 onSubmit 事件(如果没有值,则会返回一个空对象 ‘{}’)。
// demo0
import {
SchemaForm,
Submit,
SchemaMarkupField as Field
} from '@formily/antd';
<SchemaForm
components={{ Input }}
onSubmit={console.log}
>
<Field title="昵称" name="nickname" x-component="Input" />
<Submit>提交</Submit>
</SchemaForm>
表单校验
在上一个表单的基础上增加校验功能。
// demo1
import {
SchemaForm,
Submit,
SchemaMarkupField as Field
} from '@formily/antd';
<SchemaForm
components={{ Input }}
onSubmit={console.log}
>
{/* 只需要一个必填校验,增加required属性即可,这时message提示为 “该字段是必填字段” */}
<Field required title="昵称" name="nickname0" x-component="Input" />
{/* 自定义message则需要使用 x-rules,属性:ValidatePatternRules */}
<Field
title="昵称"
name="nickname1"
x-component="Input"
x-rules={{ required: true, message: "昵称不能为空" }}
/>
{/* x-rules 支持数组,可以进行多个判断 */}
<Field
title="url"
name="url"
x-component="Input"
x-rules={[
{ required: true, message: "url不能为空" },
{ format: "url", message: "url格式有误" },
/* 自定义校验函数:CustomValidator:() => ValidateResponse */
(value, description, rules) => {
console.log(value, description, rules);
return {
type: 'error',
message: "string"
}
}
]}
/>
<Submit>提交</Submit>
</SchemaForm>
自定义校验正则
自定义正则校验,需要先使用registerValidationFormats注册,然后在x-rules内使用。
// demo2
import {
SchemaForm,
Submit,
SchemaMarkupField as Field,
registerValidationFormats
} from '@formily/antd';
// 内置format正则:InternalFormats
registerValidationFormats({
integer: /^d+$/
});
<SchemaForm
components={{ Input }}
onSubmit={console.log}
>
<Field
title="电话"
name="phoneNumber"
x-component="Input"
x-rules={{ format: "integer", message: "请输入纯数字" }}
/>
<Submit>提交</Submit>
</SchemaForm>
常用表单示例
官方示例
// demo3
import { Input, InputNumber, Select, DatePicker } from "antd";
import { SchemaForm, Submit, SchemaMarkupField as Field } from "@formily/antd";
import { Range, Checkbox, Radio } from "@formily/antd-components";
<SchemaForm
components={{
Input,
TextArea: Input.TextArea,
Password: Input.Password,
InputNumber,
DatePicker,
RangePicker: DatePicker.RangePicker,
Checkbox,
CheckboxGroup: Checkbox.Group,
Radio,
RadioGroup: Radio.Group,
Select,
Range
}}
onSubmit={console.log}
>
<Field title="String" name="string" x-component="Input" />
<Field title="Password" name="password" x-component="Password" />
<Field
title="InputNumber"
name="inputNumber"
x-component="InputNumber"
/>
<Field title="TextArea" name="textarea" x-component="TextArea" />
<Field
title="Simple Select"
name="simpleSelect"
x-component="Select"
enum={["1", "2", "3", "4"]}
/>
<Field
title="Object Select"
name="objSelect"
x-component="Select"
enum={[
{ label: "One", value: "1" },
{ label: "Two", value: "2" },
{ label: "Three", value: "3" },
{ label: "Four", value: "4" }
]}
/>
<Field title="Switch" name="switch" x-component="Switch" />
<Field title="DatePicker" name="datePicker" x-component="DatePicker" />
<Field
title="RangePicker"
name="[start,end]"
x-component="RangePicker"
/>
<Field
title="Range"
name="range"
x-component="Range"
x-component-props={{
min: 0,
max: 1024,
marks: [0, 1024]
}}
/>
<Field
title="Simple Checkbox"
name="simpleCheckbox"
x-component="CheckboxGroup"
enum={["1", "2", "3", "4"]}
/>
<Field
title="Object Checkbox"
name="objectCheckbox"
x-component="CheckboxGroup"
enum={[
{ label: "One", value: "1" },
{ label: "Two", value: "2" },
{ label: "Three", value: "3" },
{ label: "Four", value: "4" }
]}
/>
<Field
title="Simple Radio"
name="simpleRadio"
x-component="RadioGroup"
enum={["1", "2", "3", "4"]}
/>
<Field
title="Object Radio"
name="objectRadio"
x-component="RadioGroup"
enum={[
{ label: "One", value: "1" },
{ label: "Two", value: "2" },
{ label: "Three", value: "3" },
{ label: "Four", value: "4" }
]}
/>
<Submit>提交</Submit>
</SchemaForm>
Interfaces
export type InternalFormats =
| 'url'
| 'email'
| 'ipv6'
| 'ipv4'
| 'idcard'
| 'taodomain'
| 'qq'
| 'phone'
| 'money'
| 'zh'
| 'date'
| 'zip'
| string
export type ValidatePatternRules =
| InternalFormats
| CustomValidator
| ValidateDescription
| ValidateArrayRules
export type CustomValidator = (
value: any,
description?: ValidateDescription,
rules?: ValidateRulesMap
) => ValidateResponse
export type ValidateResponse = SyncValidateResponse | AsyncValidateResponse
欢迎大家留言,可以告诉我你不清楚的地方以及你的其他见解 🙂